Manual - PDF417
Introduction
PDF417 code comes from the 2D barcode family. You can encode a large amount of information within a single barcode.
All the ASCII characters from 0 to 255 are supported. PDF417 encodes the data differently based on the type of characters provided. Some characters are encoded with a higher compression level. See the compression level on the technical page.
This class inherits the BCGBarcode2D class.
Example
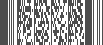
Methods
BCGpdf417's Methods
- getQuietZone() — Gets if the quiet zone will be drawn
-
setQuietZone(
$quietZone ) — Specifies to draw a quiet zone around the barcode - getCompact() — Gets if the barcode will be compact/truncated
-
setCompact(
$compact ) — Specifies if the barcode must be compact/truncated - getColumn() — Gets the number of data column
-
setColumn(
$column ) — Sets the number of data column - getErrorLevel() — Gets the error correction level of the barcode
-
setErrorLevel(
$level ) — Sets the error correction level of the barcode - getRatio() — Gets the ratio for printing
-
setRatio(
$ratio ) — Sets the ratio for printing
BCGBarcode2D's Methods
-
setScaleX(
$scaleX ) — Sets the scaling X for the barcode -
setScaleY(
$scaleY ) — Sets the scaling Y for the barcode
BCGBarcode's Methods
-
parse(
$text ) — Analyzes a$text message to draw afterwards -
draw(
$image ) — Draws the barcode on the$image -
getDimension(
$width ,$height ) — Returns an array containing the required size for the image - getScale() — Gets the scale of the barcode
-
setScale(
$scale ) — Sets the scale of the barcode - getForegroundColor() — Gets the color of the bars
-
setForegroundColor(
$color ) — Sets the color of the bars - getBackgroundColor() — Gets the color of the spaces
-
setBackgroundColor(
$color ) — Sets the color of the spaces -
setColor(
$foregroundColor ,$backgroundColor ) — Sets the color of the bars and spaces - getOffsetX() — Gets the X offset
-
setOffsetX(
$value ) — Sets the X offset - getOffsetY() — Gets the Y offset
-
setOffsetY(
$value ) — Sets the Y offset -
addLabel(
$label ) — Adds a label to the graphic -
removeLabel(
$label ) — Removes a label from the graphic - clearLabels() — Removes the labels from the graphic
Code Example
<?php
use BarcodeBakery\Common\BCGColor;
use BarcodeBakery\Common\BCGDrawing;
use BarcodeBakery\Barcode\BCGpdf417;
$colorBlack = new BCGColor(0, 0, 0);
$colorWhite = new BCGColor(255, 255, 255);
// Barcode Part
$code = new BCGpdf417();
$code->setScale(3);
$code->setForegroundColor($colorBlack);
$code->setBackgroundColor($colorWhite);
$code->setErrorLevel(2);
$code->setCompact(false);
$code->setQuietZone(true);
$code->parse('PDF417');
// Drawing Part
$drawing = new BCGDrawing($code, $colorWhite);
header('Content-Type: image/png');
$drawing->finish(BCGDrawing::IMG_FORMAT_PNG);
?>
Method explanations
-
getQuietZone()
—
Gets if the quiet zone will be drawn
Returns
bool -true if activated,false otherwise -
setQuietZone(
$quietZone ) — Specifies to draw a quiet zone around the barcodeDescriptionTo be read correctly, the PDF417 must have a quiet zone around the image. You can, however, turn this off.
The default value is true. -
getCompact()
—
Gets if the barcode will be compact/truncated
DescriptionGets if the barcode will be compact/truncated.Returns
bool -true if compact/truncated -
setCompact(
$compact ) — Specifies if the barcode must be compact/truncatedDescriptionCuts the barcode by removing the 2 last columns on the right. Your barcode will be smaller but harder to read.
The default value is false. -
getColumn()
—
Gets the number of data column
DescriptionGets the number of columns.Returns
int -
setColumn(
$column ) — Sets the number of data columnDescriptionSpecifies the number of data columns you want to write. You can spread your data more horizontally than vertically by modifying this parameter.
The number of columns must be between 1 and 30.
Setting the value to -1 makes the barcode calculate this number automatically and optimize it.
The default value is -1. -
getErrorLevel()
—
Gets the error correction level of the barcode
DescriptionGets the error level.Returns
int -
setErrorLevel(
$level ) — Sets the error correction level of the barcodeDescriptionThis is the error correction level which allows you to detect and correct errors in the barcode.
The level must be between 0 and 8.
Setting this value calculates the error level automatically by trying to obtain optimal error detection.
The number of keywords you write will be restricted depending on the error level you choose. See the technical page for more information.
The default value is -1. -
getRatio()
—
Gets the ratio for printing
DescriptionGets the ratio for printing.Returns
int -
setRatio(
$ratio ) — Sets the ratio for printingDescriptionSets the ratio for printing. This is used only if the number of columns is chosen automatically since this parameter will affect the number of columns in your barcode.
If the number is under 1, the barcode will be spread more horizontally.
The default value is -1.
-
setScaleX(
$scaleX ) — Sets the scaling X for the barcodeDescriptionThe width in pixel of one module.
The default value is 1.
Note that this method is public. -
setScaleY(
$scaleY ) — Sets the scaling Y for the barcodeDescriptionThe height in pixel of one module.
The default value is 1.
Note that this method is public.
-
parse(
$text ) — Analyzes a$text message to draw afterwardsDescriptionThe data you pass to the$text argument must be supported by the type of barcode you use.
Check each barcode's introduction section to obtain more information on how to use this method within each symbology. -
draw(
$image ) — Draws the barcode on the$image DescriptionThe value of the$image argument must be an image resource. The size of the image can be defined by the value received fromgetDimension() . -
getDimension(
$width ,$height ) — Returns an array containing the required size for the imageDescriptionReturns an array in which the first index is the image width and the second index is the image height.
The arguments are used to specify the starting point of the drawing. Should be 0 for both.
TheBCGDrawing class uses this method to create the image resource.Returnsarray(int, int) - [0] is the width, [1] is the height -
getScale()
—
Gets the scale of the barcode
DescriptionGets the scale of the barcode. The value is the number of the "smallest" unit in pixel.Returns
int - value in pixels -
setScale(
$scale ) — Sets the scale of the barcodeDescriptionThe barcode will be$x times bigger. Then a pixel will be$x by$x for its size. -
getForegroundColor()
—
Gets the color of the bars
DescriptionGets the color of the bars of the barcode.Returns
-
setForegroundColor(
$color ) — Sets the color of the bars -
getBackgroundColor()
—
Gets the color of the spaces
DescriptionGets the color of the spaces of the barcode.Returns
-
setBackgroundColor(
$color ) — Sets the color of the spaces -
setColor(
$foregroundColor ,$backgroundColor ) — Sets the color of the bars and spacesDescriptionAn easy and fast method to set the color of the bars and spaces. Check thesetForegroundColor() andsetBackgroundColor() . -
getOffsetX()
—
Gets the X offset
DescriptionGets the X offset of the barcode in pixels. The value isn't multiplied by the scale.Returns
int - value in pixels -
setOffsetX(
$value ) — Sets the X offsetDescriptionSpecifies the X offset of the barcode in pixels multiplied by the scale. The required size returned bygetDimension() will be modified accordingly. -
getOffsetY()
—
Gets the Y offset
DescriptionGets the Y offset of the barcode in pixels. The value isn't multiplied by the scale.Returns
int - value in pixels -
setOffsetY(
$value ) — Sets the Y offsetDescriptionSpecifies the Y offset of the barcode in pixels multiplied by the scale. The required size returned bygetDimension() will be modified accordingly. -
addLabel(
$label ) — Adds a label to the graphicDescriptionAdds aBCGLabel object to the drawing. -
removeLabel(
$label ) — Removes a label from the graphicDescriptionRemoves a specificBCGLabel object from the drawing. -
clearLabels()
—
Removes the labels from the graphic
DescriptionClears the
BCGLabel objects from the drawing.